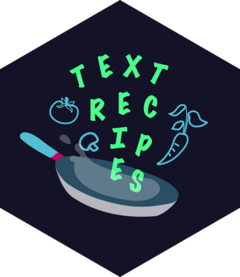
Sentencepiece Tokenization of Character Variables
Source:R/tokenize_sentencepiece.R
step_tokenize_sentencepiece.Rd
step_tokenize_sentencepiece()
creates a specification of a recipe step
that will convert a character predictor into a token
variable using SentencePiece tokenization.
Arguments
- recipe
A recipes::recipe object. The step will be added to the sequence of operations for this recipe.
- ...
One or more selector functions to choose which variables are affected by the step. See
recipes::selections()
for more details.- role
Not used by this step since no new variables are created.
- trained
A logical to indicate if the quantities for preprocessing have been estimated.
- columns
A character string of variable names that will be populated (eventually) by the
terms
argument. This isNULL
until the step is trained byrecipes::prep.recipe()
.- vocabulary_size
Integer, indicating the number of tokens in the final vocabulary. Defaults to 1000. Highly encouraged to be tuned.
- options
A list of options passed to the tokenizer.
- res
The fitted
sentencepiece::sentencepiece()
model tokenizer will be stored here once this preprocessing step has be trained byrecipes::prep.recipe()
.- skip
A logical. Should the step be skipped when the recipe is baked by
recipes::bake.recipe()
? While all operations are baked whenrecipes::prep.recipe()
is run, some operations may not be able to be conducted on new data (e.g. processing the outcome variable(s)). Care should be taken when usingskip = FALSE
.- id
A character string that is unique to this step to identify it.
Value
An updated version of recipe
with the new step added
to the sequence of existing steps (if any).
Details
If you are running into errors, you can investigate the progress of the
compiled code by setting options = list(verbose = TRUE)
. This can reveal if
sentencepiece ran correctly or not.
Tidying
When you tidy()
this step, a tibble is returned with
columns terms
and id
:
- terms
character, the selectors or variables selected
- id
character, id of this step
See also
step_untokenize()
to untokenize.
Other Steps for Tokenization:
step_tokenize()
,
step_tokenize_bpe()
,
step_tokenize_wordpiece()
Examples
library(recipes)
library(modeldata)
data(tate_text)
tate_rec <- recipe(~., data = tate_text) |>
step_tokenize_sentencepiece(medium)
tate_obj <- tate_rec |>
prep()
bake(tate_obj, new_data = NULL, medium) |>
slice(1:2)
#> # A tibble: 2 × 1
#> medium
#> <tknlist>
#> 1 [12 tokens]
#> 2 [3 tokens]
bake(tate_obj, new_data = NULL) |>
slice(2) |>
pull(medium)
#> <textrecipes_tokenlist[1]>
#> [1] [3 tokens]
#> # Unique Tokens: 3
tidy(tate_rec, number = 1)
#> # A tibble: 1 × 2
#> terms id
#> <chr> <chr>
#> 1 medium tokenize_sentencepiece_hWz4c
tidy(tate_obj, number = 1)
#> # A tibble: 1 × 2
#> terms id
#> <chr> <chr>
#> 1 medium tokenize_sentencepiece_hWz4c